Validating Email Addresses in Python
rajneesh
6 min read
- react
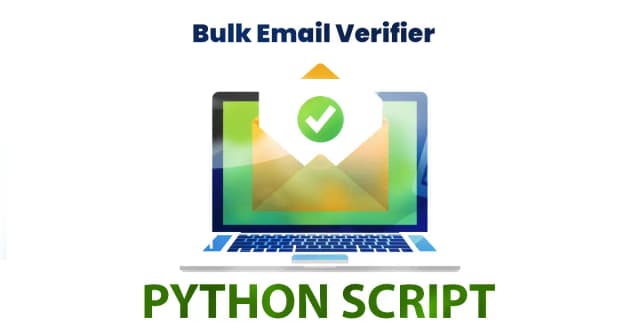
Are you looking for a method by which you can validate the email addresses?
after reading this post, you can easily validate which email is valid or not. I am going to provide a Python script by which you can validate an email or list of emails with one click. When we are doing email marketing or any other work where we have a lot of emails, but we don't know whether all the emails are valid or not, because we know that email marketing is a costly process and to save money, we should have only the correct emails so that we can do our further work. also we can reduce the eliminate hard bounces, reduce spam complaints and avoid getting blacklisted.
What is Email Validation?
Email verification is a most important process that confirms the validity and deliverability of an email address: it is commonly used by businesses to ensure the user reponse and reduce the spam complaints.This procedure will catch if there are any typos or other mistakes which might result in misdirects and bounces. Email validation will also confirm whether the email exists with a valid domain or not such as Google or Yahoo.
What You'll Need
Before we dive into the to understand the code, make sure you have the following:
Python Installed: Download and install Python from python.org.
editor: use any text editor like notepad or vs code.
The Email Validation Script
Here's the Python code we'll be working with that. if you don't understand everything right know don`t worry beacuse we are going to breaking down it step-by-step:
import re
import smtplib
import dns.resolver
import argparse
import os
def is_valid_email(email):
regex = r'^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$'
return re.match(regex, email) is not None
def get_mx_records(domain):
try:
records = dns.resolver.resolve(domain, 'MX')
mx_records = [record.exchange.to_text() for record in records]
return mx_records
except Exception as e:
print(f"Error retrieving MX records: {e}")
return None
def verify_email_smtp(email, mx_records):
try:
domain = email.split('@')[1]
mx_server = mx_records[0]
server = smtplib.SMTP()
server.set_debuglevel(0)
server.connect(mx_server)
server.helo(server.local_hostname)
server.mail('test@example.com')
code, message = server.rcpt(email)
server.quit()
return code == 250
except Exception as e:
print(f"Error verifying email: {e}")
return False
def check_email_deliverability(email):
if not is_valid_email(email):
return "Invalid email format."
domain = email.split('@')[1]
mx_records = get_mx_records(domain)
if not mx_records:
return "MX records not found."
if verify_email_smtp(email, mx_records):
return "Email is deliverable."
else:
return "Email is not deliverable."
def validate_emails(emails, check_deliverability):
results = {}
for email in emails:
if check_deliverability:
results[email] = check_email_deliverability(email)
else:
results[email] = "Valid email format" if is_valid_email(email) else "Invalid email format"
return results
def read_emails_from_file(file_path):
if not os.path.isfile(file_path):
print(f"File not found: {file_path}")
return None
with open(file_path, 'r') as file:
emails = file.read().splitlines()
return emails
def main():
parser = argparse.ArgumentParser(description="Email validation tool")
parser.add_argument('-e', '--email', type=str, help="Email address to validate")
parser.add_argument('-f', '--file', type=str, help="Path to file containing email addresses to validate")
args = parser.parse_args()
if args.email or args.file:
check_deliverability = input("Do you want to check email deliverability? (yes/no): ").strip().lower() == 'yes'
if args.email:
if check_deliverability:
result = check_email_deliverability(args.email)
else:
result = "Valid email format" if is_valid_email(args.email) else "Invalid email format"
print(f"{args.email}: {result}")
elif args.file:
emails = read_emails_from_file(args.file)
if emails:
results = validate_emails(emails, check_deliverability)
for email, result in results.items():
print(f"{email}: {result}")
else:
print("Please provide an email address or a file path containing email addresses.")
if __name__ == "__main__":
main()
important note:- If you are not interested in knowing how this script is working then you can directly go to the line how to run this script
Code Breakdown
note:- to undertand this code you must have know the basic knowledge of python
1. Validating Email Format
The is_valid_email
function is use to check is it validate email format or not:
def is_valid_email(email):
regex = r'^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$'
return re.match(regex, email) is not None
2. Retrieving MX Records
The get_mx_records
function retrieves the MX (Mail Exchange is indicates how email messages should be routed in accordance with the Simple Mail Transfer Protocol) records for the email domain:
def get_mx_records(domain):
try:
records = dns.resolver.resolve(domain, 'MX')
mx_records = [record.exchange.to_text() for record in records]
return mx_records
except Exception as e:
print(f"Error retrieving MX records: {e}")
return None
3. Verifying Email via SMTP
The verify_email_smtp
function is use to verify SMTP email address is deliverable or not:
def verify_email_smtp(email, mx_records):
try:
domain = email.split('@')[1]
mx_server = mx_records[0]
server = smtplib.SMTP()
server.set_debuglevel(0)
server.connect(mx_server)
server.helo(server.local_hostname)
server.mail('test@example.com')
code, message = server.rcpt(email)
server.quit()
return code == 250
except Exception as e:
print(f"Error verifying email: {e}")
return False
4. Checking Email Deliverability
The check_email_deliverability
function integrates the above functions to check if an email is deliverable:
def check_email_deliverability(email):
if not is_valid_email(email):
return "Invalid email format."
domain = email.split('@')[1]
mx_records = get_mx_records(domain)
if not mx_records:
return "MX records not found."
if verify_email_smtp(email, mx_records):
return "Email is deliverable."
else:
return "Email is not deliverable."
5. Validating Multiple Emails
The validate_emails
function validates a list of emails, checking their format and optionally their deliverability:
def validate_emails(emails, check_deliverability):
results = {}
for email in emails:
if check_deliverability:
results[email] = check_email_deliverability(email)
else:
results[email] = "Valid email format" if is_valid_email(email) else "Invalid email format"
return results
6. Reading Emails from a File
The read_emails_from_file
function reads email addresses from a file:
def read_emails_from_file(file_path):
if not os.path.isfile(file_path):
print(f"File not found: {file_path}")
return None
with open(file_path, 'r') as file:
emails = file.read().splitlines()
return emails
7. Command-Line Interface
The main
function provides a command-line interface to use the email validation tool:
def main():
parser = argparse.ArgumentParser(description="Email validation tool")
parser.add_argument('-e', '--email', type=str, help="Email address to validate")
parser.add_argument('-f', '--file', type=str, help="Path to file containing email addresses to validate")
args = parser.parse_args()
if args.email or args.file:
check_deliverability = input("Do you want to check email deliverability? (yes/no): ").strip().lower() == 'yes'
if args.email:
if check_deliverability:
result = check_email_deliverability(args.email)
else:
result = "Valid email format" if is_valid_email(args.email) else "Invalid email format"
print(f"{args.email}: {result}")
elif args.file:
emails = read_emails_from_file(args.file)
if emails:
results = validate_emails(emails, check_deliverability)
for email, result in results.items():
print(f"{email}: {result}")
else:
print("Please provide an email address or a file path containing email addresses.")
if __name__ == "__main__":
main()
how to run this script
Step-by-Step Instructions
1. Install Required Libraries
You need to install a some python modules to make this script work. Open your command prompt (CMD) or terminal and run:
pip install dnspython
2. Copy the Script
copy the entire script complete code provided above and paste it into your text editor. Save the file as email_validator.py
3. Run the Script
open your command prompt or terminal, navigate to the those directory where you saved email_validator.py
, and run the script by typing:
python email_validator.py -e youremail@example.com
Replace youremail@example.com
with the email address you want to validate.
4. Validate Multiple Emails from a File
If you have multiple email addresses then , save those emails in a text file (e.g., emails.txt
), with each email on a new line. Then, run:
python email_validator.py -f emails.txt
conclusion
if you to email marketing then this script is very useful for you. most of the company charge of loot of money for validate the emails i have provide a free python script by which do this in one click. if you know python then you can update this script according to you need or you can create your own website for email validate by which you can earn money. make sure before running this script please install the the required modules. share this post someone who need this script. if you want more script like this you can comment it i will create that of script also. to get notification please login or allow notification to stay updated.