Understanding the Double Exclamation Mark (!!) in JavaScript
rajneesh
3 min read
- javascript
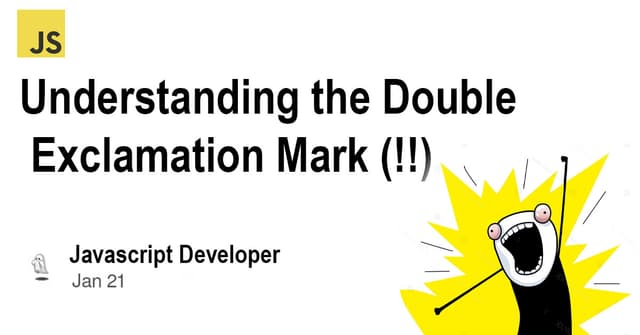
As a JavaScript developer, you might encounter with !!
operator its known as the double exclamation mark. This operator might seem confusing at first, but it’s actually quite simple once you understand its purpose: to convert any value into a boolean. first time, i will also confused that what it actually do. I will explain this operator in very simple words with examples.
What Does !!
Do?
In JavaScript, the double exclamation mark is use to convert value to Boolean. It ensure that the value is either true
or false
. it's a shorthand property to caste the value to Boolean data type.
How Does It Work?
To understand how !!
works, let's us find out first how single exclamation mark (!
) work. The !
operator is known as the logical NOT operator. It inverts the truthiness of a value:
!true
becomesfalse
!false
becomestrue
When you apply a single exclamation mark to a value, it converts the value into boolean expiration after that it invert the value. here is the example of single exclamation mark.
console.log(!true); // Output: false
console.log(!false); // Output: true
console.log(!1); // Output: false (1 is true)
console.log(!0); // Output: true (0 is false)
Now, if you apply one more single exclamation mark to the result, then over result agent invert it back to its original boolean state:
console.log(!!true); // Output: true
console.log(!!false); // Output: false
console.log(!!1); // Output: true
console.log(!!0); // Output: false
Why Use !!
?
The main reason to use !!
is to forcefully convert any value of data type into boolean data. This is very useful where you want to ensure that the value is strictly true
or false
:
Checking Existence: use can use this to check the data not be
null
norundefined by using this method
example:
let user = { name: "Alice", age: 30 }; console.log(!!user.name); // Output: true console.log(!!user.address); // Output: false
Simplifying Conditional Checks: It simplifies and clarifies the intention an of condition checks:
let isLoggedIn = !!userSession; if (isLoggedIn) { // User is logged in } else { // User is not logged in }
Type Conversion: its help you to ensure that the you are passing right data to the function it is more helpful in typescript:
function process(isValid) {
if (isValid) {
console.log("Processing...");
} else {
console.log("Invalid input");
}
}
let input = "some string";
process(!!input); // Output: Processing...
Conclusion
it is a golden method for all the coder who code in typescript. The double exclamation mark (!!
) is a very easy method to convert the value into boolean. by using to simple operator. if you are beginner in JavaScript you might encounter with this operator anytime 😅. its help you to write clean and more predictable code. especially when dealing with conditional logic and type conversions. if you think its helpful for you you can share this post with you fellow friends and if you think i missed any topic related to this operator then you can comment it i will defiantly add this on my post.