Understanding Python’s Try Except Block
rajneesh
4 min read
- python
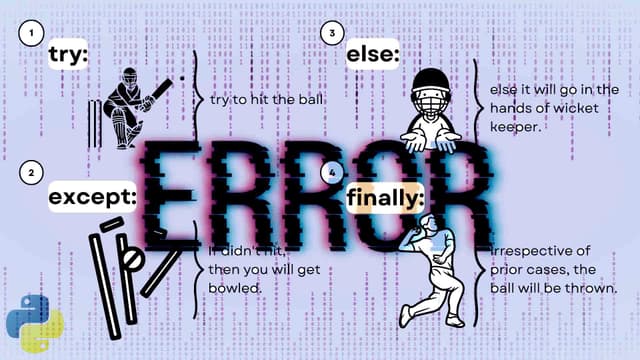
Python is a very popular programming language because it’s easy to read and write. as a professional python developer you need to thing about the error as well. to handle the error in python. python provided a powerful and user-friendly error-handling mechanism, specifically the try
and except
blocks. for this method i have followed many professional python developer GitHub repositories by that knowledge i will explain this topic in very easy words. Let’s dive into the try
and except
blocks, which are Python’s tools for error handling.
What Are Exceptions?
In Python, an exception is an event that occurs during the execution of a program that disrupts the normal flow of the program’s execution. In simple terms, it’s an error that happens while the program is running. Unlike syntax errors, which are mistakes in the program’s code that prevent it from running at all, exceptions can occur even if the code is syntactically correct.
The Try Block
The try
block lets you test a block of code for errors. it means that try block check or you can say test the code inside the try block its error free or not. here is the code structure :
try:
# Code that might cause an exception
x = 10 / 0
f = open("demofile.txt", "rt")
When Python executes this code, if anything goes wrong within the try
block (like trying to open a non-existent file or dividing by zero, you can see example above), it stops executing the try
block and moves to the except
block.
The Except Block
The except
block will handle the error. You can specify handle the different types of exceptions to catch different kinds of errors here is some example:
try:
# Code that might cause an exception
x = 10 / 0
f = open("demofile.txt", "rt")
print(f.read())
except FileNotFoundError:
# Code to handle the file not found error
print("File not found. Check the path variable and filename")
except ZeroDivisionError:
# Code to handle dividing by zero
print("not divisible by zero")
except Exception as e:
# Code to handle any other exception
print(f"An error occurred: {e}")
If an exception occurs, the type of exception is shown, and the corresponding except
block is executed (in above code if file not found error occur then FileNotFoundError block run like this according to their error type except block executed) . If no exception occurs, the except
block is skipped.
Else and Finally
here are two other blocks that can be used with try
and except
:
else
: This block is executed if no exceptions will occur in thetry
block.finally
: This block is executed no matter what, and is typically used for cleaning up resources, like closing files.
Here’s how they look in code:
try:
# Code that might cause an exception
f = open("demofile.txt", "rt")
print(f.read())
except Exception as e:
# Code to handle the exception
else:
# Code to execute if no exceptions
print("file reeded successfully")
finally:
# Code that is always executed
f.close()
Raising Exceptions
Sometimes, you might want to raise an exception intentionally if a certain condition is not met. You can use the raise
keyword for this example:
x = -1
if x < 0:
raise Exception("Sorry, no numbers below zero")
Conclusion
Using try
and except
blocks in Python helps you deal with errors. you can say that it is an advance topic of python programming because by this you can learn one of the most usable concept of programming. it move you one more step of learning python. it is a good practice to use try except block. its make you code user-friendly. if you like this post don't forget to like share and follow me on this website. if you have a interest python programming you can read my more post related to python. the key point of professional python developer is not just write a working code. write code that handles the unexpected error that way by which any one understand you code.