Understanding JavaScript setTimeout
ASHISH GUPTA
3 min read
- JavaScript
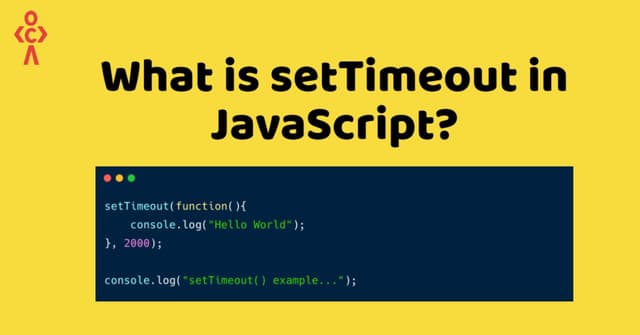
while creating any project we need to execute to any function or method after some interval of time that time we can use javascript setTimeout function.
its help you to mange time of function execute. One of the key functions that helps with this is setTimeout
. In this blog post we'll cover what is setTimeout
?, how to implement or write code effectively, and how you can effectively use it in your projects.
What is setTimeout
?
setTimeout
is a built-in JavaScript function that help you to run a function after a given time interval in millisecond
Syntax
setTimeout(function, delay);
How setTimeout
Works
setTimeout is take two parameter first is function that you want to execute after that delay. and second parameter how much delay you need to required to execute that function in milliseconds.
Example
setTimeout(() => {
console.log('This function will execute after 2 seconds');
}, 2000);
Practical Use Cases
Delaying Execution
sometime we need to show notification allow permission modal to user to ask user to allow notification for your website. you can see some example on this site after some time of interval a popup notification modal open that function will also created by setTimeout function. here the simple code example:
setTimeout(() => {
alert('allow notification, after 5 seconds');
}, 5000);
Managing Asynchronous Code
setTimeout
can help manage asynchronous code by deferring actions until after certain tasks are completed. For example,when we need to execute any api call after some time of interval while any component render.
function asyncOperation() {
setTimeout(() => {
console.log('Asynchronous operation completed');
}, 2000);
}
asyncOperation(); //call this function after that component or element render.
Advanced Techniques
Passing Arguments to the Callback Function
You can pass additional parameters to the callback function using setTimeout
. 3rd parameter which pass as a argument to the callback function.
setTimeout((message) => {
console.log(message);
}, 2000, 'Hello, World!');
Clearing a Timeout
If you need to cancel a scheduled setTimeout
, you can use clearTimeout
.
const timeoutId = setTimeout(() => {
console.log('This will not be logged');
}, 2000);
clearTimeout(timeoutId);
in upper code you can see that clearTimeout function that while cancel the timoutId function for execution.
Common Pitfalls and Best Practices
Common Mistakes
Forgetting to clear a timeout: Always clear timeouts when they are no longer needed to avoid unexpected behavior.
Using incorrect delay values: Ensure the delay is specified in milliseconds.
Best Practices
Always clear timeouts when they are no longer needed: This can help prevent memory leaks and other issues in your code.
Use meaningful delay values: Choose delay values that make sense for your application's user experience
Comparison with Other Timing Functions
setInterval
Unlike setTimeout
, setInterval
repeatedly executes the callback function at specified intervals back to back.
const intervalId = setInterval(() => {
console.log('This message is logged every 2 seconds');
}, 2000);
requestAnimationFrame
This function is more suitable for animations as it syncs with the browser's repaint cycle. It provides a way to optimize animations for performance.
function animate() {
console.log('Animation frame');
requestAnimationFrame(animate);
}
requestAnimationFrame(animate);
conclusion
in this blog post i have covered all the important points related to this topic with comparison with other type of settime function with suitable examples if you are beginner or advance developer of java script any one easily understand this post and master the settimeout function easily. i have explain settimeout function with suitable examples by which easily understand this function and used in your real life project easily.