Python's One-Line if else Magic
rajneesh
3 min read
- python
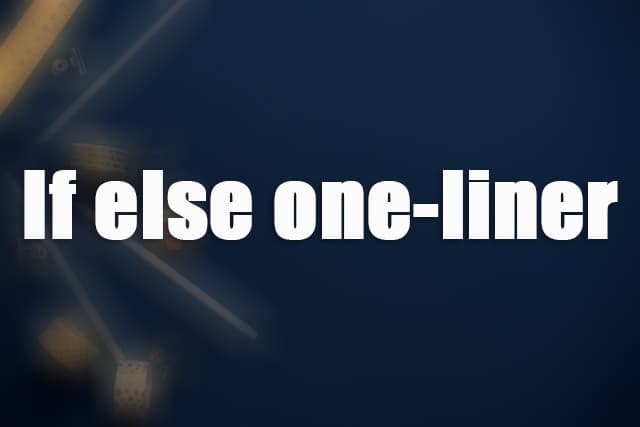
are you looking for a method by which you can write the if else statement in one Line? then you have come to the right post.
i am 100% sure that with this method, your code will become clean, simple and easy to understand, like a professional code.
To find this method, i looked at the python official documentation as well as several GitHub open source repositories like
Why Use One-Line If-Else?
its makes the code clear, shorter and easier to understand
one-liner increase the code readability and also highlight the logic of the code without unnecessary indentation or verbosity.
its make the code more effective by reducing the code lines
Syntax of One-Line If-Else
syntax for one-line if-else statement in python
value_if_true if condition else value_if_false
the structure of one line is "value_if_true"
if condition
is true, otherwise "value_if_false"
will its false.
example:
x = 10
result = "Even" if x % 2 == 0 else "Odd"
print(result) # Output: "Even"
In this example:
x % 2 == 0
is the condition for this statement .if condition is true than it return "Even"
if condition is false than it return "Odd"
if else of one-line with list, tuple, string
[expression for item in iterable if condition]
expression
: The expression is the current item of the iteration, which can be manipulated before into the new list.item
: The each value of the iterable received in the item if condition is true.iterable
: The sequence (e.g., list, tuple, string) to iterate over.condition
(optional): it is an optional filter condition for this expression
Example:
#without condition
squares = [x**2 for x in range(1, 6)]
print(squares) # Output: [1, 4, 9, 16, 25]
#with condition
# Filter even numbers from a list
numbers = [1, 2, 3, 4, 5]
even_numbers = [num for num in numbers if num % 2 == 0]
print(even_numbers) # Output: [2, 4]
In the above example:
[x**2 for x in range(1, 6)]
creating new list of square numbers by using List Comprehension .[num for num in numbers if num % 2 == 0]
filters out the even number for the list by condition option.
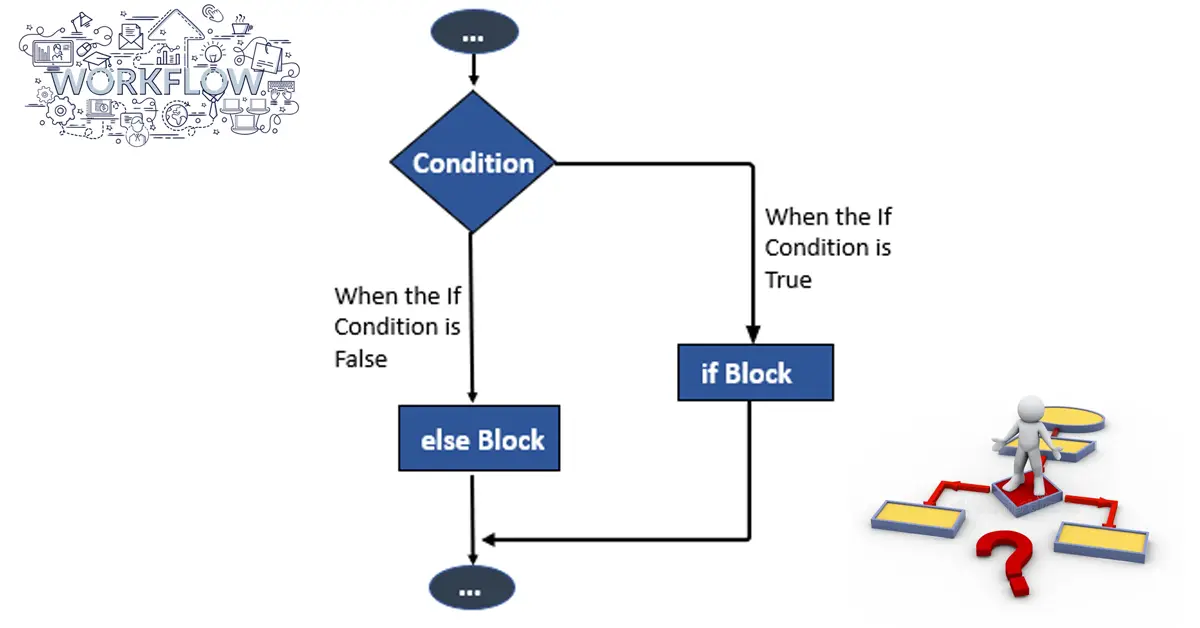
Dictionary Assignment
we can do this same thing with dictionary
Syntax:
{key_expression: value_expression for item in iterable if condition}
key_expression
: The expression used to get key for dictionaryvalue_expression
: The expression used to get value for dictionary.item
: The each value of the iterable received in the item if condition is true.iterable
: The sequence to iterate over (e.g., list, tuple).condition
(optional): it is an optional filter condition for this expression
Example:
#without condition
# Create a dictionary of squares of numbers from 1 to 5
squares_dict = {x: x**2 for x in range(1, 6)}
print(squares_dict) # Output: {1: 1, 2: 4, 3: 9, 4: 16, 5: 25}
# Filter and create a dictionary of even numbers from a list
numbers = [1, 2, 3, 4, 5]
even_numbers_dict = {num: num for num in numbers if num % 2 == 0}
print(even_numbers_dict) # Output: {2: 2, 4: 4}
In the example above:
{x: x**2 for x in range(1, 6)}
creates a new dictionary with key and value.the key is numbers from 1 to 5 and value is squares of key.{num: num for num in numbers if num % 2 == 0}
creates a new dictionary of even number where conditions isnum % 2 == 0.
Conclusion
Python's one-line if-else statements is a best method to make your code clear and easy to readable its make your code professional. if you like my post please like share and subscribe .try this method in your code and tell me its helps or not.