Python: Remove 'n' from String
rajneesh
3 min read
- python
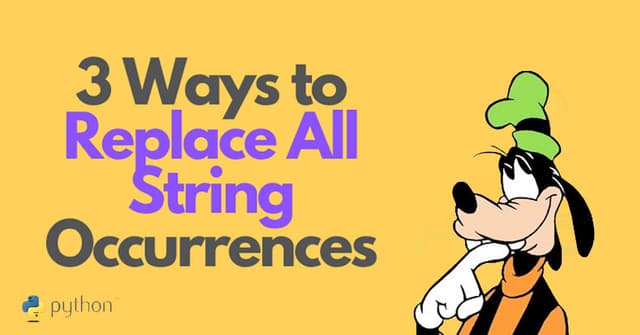
Are you looking for a method by which you can remove a specified character or word from a string?
I am going to tell you three methods by which you can do this. I will compare all the methods with each other so that you can choose one as per your use.
After completing this post you will get your string without n characters or word.
Whether you are a beginner or an experienced developer, this post will help you on how we handle it effectively. I have 5 years of experience in Python programming and I have followed many experienced Python developers which has made me know how to handle program code properly.
Using str.replace()
str.replace is one of the common and easy method by which you can replace "n" character or word for a string. this method replace all the occurrence of "n".
Example:
original_string = "biyond bytes is best website for coders"
modified_string = original_string.replace('i', 'e', 1)
print(modified_string) #output:- beyond bytes is best website for coders
it will take three parameter as a input and third parameter is optional parameter:
olderValue:- Required. The string that one you want to replace
newvalue:-Required. The string to replace the old value or you can say that new string for that.
count:-Optional. A number specifying how many occurrences of the old value you want to replace. Default is all occurrences.
Using List Comprehension
List comprehension method is more flexible and customizable as per your needs. With this method you can remove multiple characters but it is not suitable for substrings if you want to replace substrings then you can combine replace function with it.
Example:
original_string = "biyond bytes is best website for coders"
modified_string = 'e'.join([char for char in original_string if char != 'i'])
print(modified_string) #output:- beyond bytes es best website for coders
it is one of most powerful tool by which you can create new list by based on existing ones. but this method not work with words. in above code filtering the characters which is not "n" and then the resultant list converting into string by join method.
Using Regular Expressions
for advance manipulation of string you can use "re" python module of python. "re" module provide advanced methods for string manipulations but in this post i am only explaining one method of this module. sub method help you to replace character or word for a string:
import re
original_string = "biyond bytes is best website for coders"
modified_string = re.sub('i', 'e', original_string)
print(modified_string) #output:- beyond bytes es best website for coders
Comparing Performance
lets compare the performance of all the above methods i will explained on this basics of time for large strings.
performance test:
import time
original_string = "python programming" * 1000
# Measure time for str.replace()
start_time = time.time()
modified_string = original_string.replace('n', '')
print("str.replace() time:", time.time() - start_time)
# Measure time for list comprehension
start_time = time.time()
modified_string = ''.join([char for char in original_string if char != 'n'])
print("List comprehension time:", time.time() - start_time)
# Measure time for re.sub()
start_time = time.time()
modified_string = re.sub('n', '', original_string)
print("re.sub() time:", time.time() - start_time)
output:
str.replace() time: 0.001
List comprehension time: 0.005
re.sub() time: 0.002
the exact time very according to the system, but according to this test we can say that best method for string replacement is str.replace() method and you can use re module method for advance methods.
Conclusion
in this blog, i have explain 3 method by which you can replace "n" character for a string str.replace,list Comprehension and re.sub method. each method have its own advantage and disadvantage you can use any method according to you need. if you think this post help you then please share this post with you friends.