Pandas Reorder Coulumns || How to Reorder Columns in a Pandas DataFrame || BiyondBytes
ASHISH GUPTA
5 min read
- python
- Panda
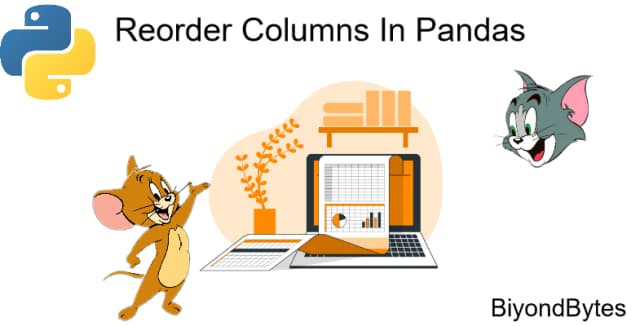
Introduction
Are you finding how to reorder columns in pandas,pandas use to create 2d-matrix and nd-matrix in python. Pandas is an crucial library for data manipulation and analysis in Python. One of the most common problem or task you may encounter is reordering the columns in a DataFrame.if you are preparing data for visualizations,reporting and further analysis manage your columns in a specific order may be very important in this blog post we'll cover various method to reorder columns in a pandas DataFrame.
Note: *All code in this post is written in python language
What is Panda's?
This Pandas is not for your girlfriend's teddy But .Pandas is a dynamic, open-source library use for data analysis and manipulation tool made use of core of the Python programming language. It provides data structures and functions needed to work on structured data seamlessly. One of its primary data structures is the DataFrame, a 2-dimensional labeled data structure with columns of potentially different types.
Installation of pandas using PIP
The installation is very simple using pip in terminal,PIP is a package manager for Python packages, or modules if you like. Note: If you have Python version 3.4 or later, PIP is included by default. in terminal just type "pip install pandas" and hit enter.
pip install pandas
Creating a Sample DataFrame
Before we go deep into reordering columns, let's create a sample DataFrame that we can work with.make first a dictionary in this dictionary you have to specify the columns name before semicolon and after semicolon write all the elements of column.
Now call a function of pandas module pd.DataFrame(your_variable),pass the variable into the function parameter.
import pandas as pd
# Creating a sample DataFrame
data = {
'A': [1, 2, 3],
'B': [4, 5, 6],
'C': [7, 8, 9],
'D': [10, 11, 12]
}
Matrix = pd.DataFrame(data)
print("Original DataFrame:")
print(Matrix)
#Output
A B C D
0 1 4 7 10
1 2 5 8 11
2 3 6 9 12
Reordering Columns Using Index of columns
Easiest ways to reorder columns is by using their index positions.
cols = list(Matrix.cols)
changing_cols = [columns[i] for i in [1, 3, 0, 2]]
Matrix = Matrix[changing_cols]
print(Matrix)
#output
B D A C
0 4 10 1 7
1 5 11 2 8
2 6 12 3 9
Reordering Columns Using Column Names
Another method is to reorder the columns by specifying their names means using columns names like D-column,B-column,etc.
# interchanging columns by name
Matrix = Matrix[['B', 'C', 'D', 'A']]
print("Matrix after reordering columns by name:")
print(Matrix)
#Output
B C D A
0 4 7 10 1
1 5 8 11 2
2 6 9 12 3
Reordering Columns by Moving a Specific Column
only move specific column in pandas ,if you want to move a specific column to a newest to it's position, by relaxing or fixing the other columns at rest of the columns in their original order.
# Moving column 'D' to the front
cols = list(Matrix.columns)
cols.insert(0, cols.pop(cols.index('D')))
Matrix= Matrix[cols]
print("DataFrame after moving column 'D' to the front:")
print(Matrix)
#output
D B A C
0 10 4 1 7
1 11 5 2 8
2 12 6 3 9
Reordering Columns with '.reindex()'
The .reindex()
method is another whippy way to reorder columns.
# Reordering columns using reindex
Matrix = Matrix.reindex(columns=['C', 'A', 'D', 'B'])
print("DataFrame after reordering columns using reindex:")
print(Matrix)
#output
C A D B
0 7 1 10 4
1 8 2 11 5
2 9 3 12 6
Using the Loc and Iloc Methods
This is the another method of reordering columns in a pandas DataFrame using the loc
and iloc
methods, which are usually used for indexing. These methods can also reorder the DataFrame by specifying the column order when selecting a subset of columns. Here's an example:
## Using loc
Matrix = Matrix.loc[:, ['B', 'D', 'A', 'C']]
## Using iloc
Matrix = Matrix.iloc[:, [1, 3, 0, 2]]
print(Matrix)
#output
D C B A
0 10 7 4 1
1 11 8 5 2
2 12 9 6 3
Moving a Specific Column to the Front or End
Moving a specific column to the front or the end of a DataFrame is a common requirement:
## Move column 'B' to the front
Matrix = Matrix[['B'] + [col for col in Matrix.columns if col != 'B']]
## Move column 'A' to the end
Matrix = Matrix[[col for col in Matrix.columns if col != 'A'] + ['A']]
print(Matrix)
#output
B C D A
0 4 7 10 1
1 5 8 11 2
2 6 9 12 3
Renaming Columns
changing names of columns in a DataFrame is easy with pandas:
Matrix = Matrix .rename(columns={'A': 'Biyond', 'B': 'Bytes', 'C': 'Beast','D': 'Youtube'})
print(Matrix )
#output
Biyond Bytes Beast Youtube
0 1 4 7 10
1 2 5 8 11
2 3 6 9 12
Swapping Multiple Columns at Once
Swapping multiple columns at once in a pandas DataFrame can be achieved with a simple technique:
Matrix = Matrix [['B', 'A'] + [col for col in Matrix .columns if col not in ['A', 'B']]]
print(Matrix)
#output
B A C D
0 4 1 7 10
1 5 2 8 11
2 6 3 9 12
Above code snippet swaps columns 'A' and 'B' in the DataFrame. It's a simple other powerful way to rearrange columns in your DataFrame without creating a new one.let's try it your own's using documentations
Conclusion
Reordering and Swapping columns in a Pandas DataFrame is a most common task and it can be done in many ways,It's totally depending on your specific needs. However you're using column indices, names, or methods like .reindex()
, Pandas make it easy to organize or manage your data exactly according to your need . Brush up these methods will help you handle data more efficiently and prepare it for any subsequent analysis or visualization. It can be use Machine learning models, data science and data analytics,
Feel free to experiment with these methods on your own datasets to get a better grasp of how they work!
Happy coding!