Merge PDF with Python: A Comprehensive Guide
rajneesh
3 min read
- react
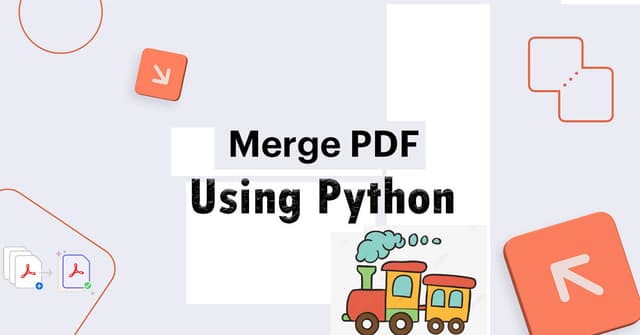
Are you looking for a method by which you can merge PDFs with Python?
I am going to provide you step by step guide that how you can merge PDF using Python code with a simple package.
After completing this guide you can get a simple Python program by which you can merge PDF in one click and by this program you can merge any PDF you want to merge.
For creating merge PDF program in Python, I have used many Python modules or packages, after using many packages I have selected the best one out of all of them.
let start,
Why Use Python for PDF Merging?
Python is a very powerful programming language. It is simple and easy to learn programming language which provides different types of modules or packages by which you can do any task 😅. Python provides lots of modules to merge PDF. After a lot of research, I have selected PyPDF2 module to merge PDF and you know coding in Python is like you are drinking water.
Prerequisites
Before starting coding please check if Python is installed or not. If Python is not installed in your PC please install it. Create a virtual environment to protect your main Python environment to prevent module pollution. Creating a virtual environment is an optional process. Then install the PyPDF2 library using this code:
pip install PyPDF2
Step-by-Step Guide to Merging PDFs
Importing required Library:- first of all import PdfMerger from PyPDF2 library:
from PyPDF2 import PdfMerger
Creating a Merger Object:- create a merger object of pdfMerger class which will you to marge all the process by this method:
merger = PdfMerger()
Appending PDF Files:- merger object provide you a append method by which add all the pdf files you want to merge by using this method in a loop here is the code:
pdf_files = ['file1.pdf', 'file2.pdf', 'file3.pdf'] for pdf in pdf_files: merger.append(pdf)
saving Merged PDF:- you can save merge file as a new pdf file with write method you can pass file name as a parameter to save pdf by that name here is the code:
merger.write("merged_document.pdf")
merger.close()
Complete Code
Here's your complete code for merging multiple PDF files:
from PyPDF2 import PdfMerger
# List of PDF files to merge
pdf_files = ['file1.pdf', 'file2.pdf', 'file3.pdf']
# Create a PdfMerger object
merger = PdfMerger()
# Append PDF files
for pdf in pdf_files:
merger.append(pdf)
# Write the merged PDF to a new file
merger.write("merged_document.pdf")
merger.close()
print("PDF files merged successfully!")
Advanced Features
PyPDF2 offers several advanced features by which you can manipulate the output of merge pdf:
Specifying Pages: by this method you can specify the pages of merge pdf of each pdf:
merger.append(file1, pages=(0, 3)) # Appends only the first three pages of file1.pdf
Inserting at Specific Positions: Insert a PDF at a specific position in the output file.
merger.merge(position=1, fileobj='file2.pdf') # Inserts file2.pdf at position 1
Conclusion
merging pdf in python is very easy due to PyPDF2 module. the capability of python is make it fully automating document management you can try your self for better understanding of python it modules. you can efficiently merge multiple PDF files by this simple code in one click. if you are from non coding background you can also easily understand this program. if you think its helpful for you. you can share this post with your friends.