JavaScript Capitalize First Letter
ASHISH GUPTA
2 min read
- JavaScript
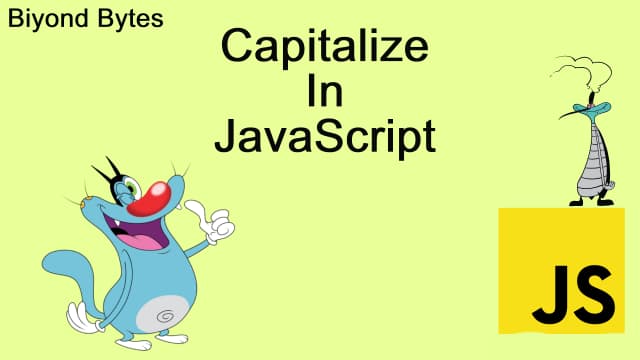
Introuduction
Hey Devs, Are you looking to capitalize your string of your first letter in JavaScript, It provides multiple methods and to modify strings. One common requirement is to capitalize the first letter of a string.If you're working on a small project or a large application, this little trick can come in handy. In this blog post, we'll learn different ways to achieve this in JavaScript.
Note : *All code is written in JavaScript you can try in your editor or in console of web browser.
Why Capitalize the First Letter?
Capitalizing the first letter of a string is useful in many scenarios, such as:
Formatting user input:it's make little bit professional UI.
Displaying titles and headings: like in this blog have headings which do automatically capitalize first letter using JavaScript.
Improving the readability of strings: it increase readability of blog or other paragraph.
Let's go deeper into the methods to capitalize the first letter of a string in JavaScript.
Method 1: Using 'charAt()'
and 'slice()'
One of the easiest ways to capitalize the first letter is by using the charAt()
and slice()
methods.
function capitalizeFirstLetter(string) {
return string.charAt(0).toUpperCase() + string.slice(1);
}
let example = "biyond bytes"
console.log(capitalizeFirstLetter(example)); // Output: Biyond bytes
Method 2: Using Template Literals
Template literals provide a more concise and readable way to achieve the same result.
function capitalizeFirstLetter(string) {
return `${string.charAt(0).toUpperCase()}${string.slice(1)}`;
}
let example = "biyond bytes";
console.log(capitalizeFirstLetter(example)); // Output: Biyond bytes
Method 3: Using Array Destructuring
Array destructuring offers another elegant solution for this task.
function capitalizeFirstLetter(string) {
const [first, ...rest] = string;
return first.toUpperCase() + rest.join('');
}
let example = "biyond bytes";
console.log(capitalizeFirstLetter(example)); // Output: Biyond bytes
Method 4: Using Regular Expressions
Regular expressions can also be used to capitalize the first letter.
function capitalizeFirstLetter(string) {
return string.replace(/^./, string[0].toUpperCase());
}
let example = "biyond bytes"
console.log(capitalizeFirstLetter(example)); // Output: Biyond bytes
Method 5: Handling Multiple Words
If you need to capitalize the first letter of each word in a string, you can use the following function:
function capitalizeWords(string) {
return string.split(' ').map(word => capitalizeFirstLetter(word)).join(' ');
}
function capitalizeFirstLetter(string) {
return string.charAt(0).toUpperCase() + string.slice(1);
}
let example = "biyond bytes"
console.log(capitalizeWords(example)); // Output: Biyond Bytes
Conclusion
Here are the methods to Capitalize the first letter of your string, in this blog have core method without using functions . you can make your own methods for capitalize the letter and we also discuss the inbuilt function in JavaScript. if you find helpful this blog please hit like button and follow me for more solution of coding minor and major problems. If you want to uppercase the whole string you can write in comment section.
Happy Coding!!