How to Download YouTube Videos as MP3 Using Python
rajneesh
4 min read
- python
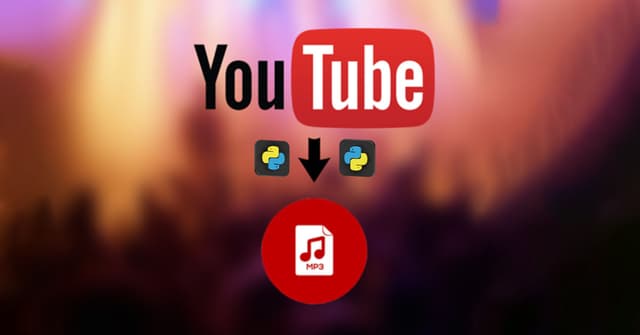
Are you looking for a method by which you can download YouTube videos as MP3 using Python?
After completing this blog post, you can get mp3 of any YouTube video with a single click.
I have tried many ways to do this, and after that, I have chosen this method which is easier and faster than any other method. You can use this Python program to create your own music playlist and extract audio from YouTube videos for lectures and podcasts. To download YouTube videos as mp3 we are using pytube library or module and os library for file manipulation.
Prerequisites
Before we begin, ensure that you have installed Python on your system. You can download it from here.
after that, you need to create env to manage your library or module. here is the blog post how you can create virtual environment. and install pytube
library on you system or env by using this command:
pip install pytube
Step-by-Step Guide
Explanation
Importing Packages
import os from pytube import YouTube from pytube.exceptions import VideoUnavailable, PytubeError
importing all the required library, methods and functions which is use to create program.
Function to Get YouTube Video Object
def get_youtube_video(url: str) -> YouTube: """sownloading youtube video audio steam and then returning as a new file""" try: return YouTube(url) except VideoUnavailable: print(f"The video at this {url} is unavailable.") raise except PytubeError as e: print(f"An error occurred: {e}") raise
Create a function for getting the url of a YouTube video and check whether the URL is available or not. if url is available then we are returning the youtube object with URL.
Function to Download Audio Stream
def download_audio_stream(yt: YouTube, destination: str = '.') -> str: """downloading youtube video audio steam and then returning as a new file""" try: audio_stream = yt.streams.filter(only_audio=True).first() out_file = audio_stream.download(output_path=destination) return out_file except PytubeError as e: print(f"An error occurred while downloading: {e}") raise
In this function, we are downloading the audio stream of the YouTube video and saving it to the specified destination. It also handles potential download errors during the downloading process.
Function to Convert File to MP3
def convert_to_mp3(file_path: str) -> str: """converting the file path into mp3 format.""" base, _ = os.path.splitext(file_path) new_file = base + '.mp3' os.rename(file_path, new_file) return new_file
This function converts the downloaded file into MP3 format by changing its extension and returning as a new file.
Main Function
def main(): url = input("Enter the URL of the video you want to download: \n>> ") yt = get_youtube_video(url) destination = input("Enter the destination (leave blank for current directory where you want to save file): \n>> ") or '.' out_file = download_audio_stream(yt, destination) mp3_file = convert_to_mp3(out_file) print(f"{yt.title} is downloaded successfully as {mp3_file}") if __name__ == '__main__': main()
main function runs the script while and mange all the function and if audio file is downloaded successfully then its print the success message on display.
complete code
here is the complete code to download youtube video as mp3 using python:
import os
from pytube import YouTube
from pytube.exceptions import VideoUnavailable, PytubeError
def get_youtube_video(url: str) -> YouTube:
"""by using youtube link create a youtube object and returning it."""
try:
return YouTube(url)
except VideoUnavailable:
print(f"The video at this {url} is unavailable.")
raise
except PytubeError as e:
print(f"An error occurred: {e}")
raise
def download_audio_stream(yt: YouTube, destination: str = '.') -> str:
"""downloading youtube video audio steam and then returning as a new file"""
try:
audio_stream = yt.streams.filter(only_audio=True).first()
out_file = audio_stream.download(output_path=destination)
return out_file
except PytubeError as e:
print(f"An error occurred while downloading: {e}")
raise
def convert_to_mp3(file_path: str) -> str:
"""converting file path into mp3 """
base, _ = os.path.splitext(file_path)
new_file = base + '.mp3'
os.rename(file_path, new_file)
return new_file
def main():
url = input("Enter url of youtube video: \n>> ")
yt = get_youtube_video(url)
destination = input("Enter the destination (leave blank for current directory where you want to save file): \n>> ") or '.'
out_file = download_audio_stream(yt, destination)
mp3_file = convert_to_mp3(out_file)
print(f"{yt.title} is downloaded successfully as {mp3_file}")
if __name__ == '__main__':
main()
Conclusion
By using this method, you can easily download YouTube videos as mp3. This library is very powerful and also provides many methods by which you can download YouTube videos in any format. With this Python program you can also earn money. You can create any website by which users can download YouTube videos as mp3 in one click and you can use Google ads to earn money. Share this program with your friends who want to create music playlists using youtube video songs by this program. Or as a video editor, you need to use music background you can download that song by this program.
if you think this post in any way then please share this post with your music lover friends ,youtube creates and video editor.